Arduino DHT11 Wifi Humidity and Temperature
You can watch DHT11 data (Humidity and Temperature) over internet/network with Android App. We use ESP8266-01 WiFi module for wireless comminication.
Required Main Parts
- Arduino
- ESP8266-01 WiFi module (If you need update your ESP8266 Firmware please check we ESP8266 Firmware update page)
- DHT11 Humidity and Temperature sensor
- Any Android device
Project Details
We use ESP8266-01 WiFi module for wireless comminication. We need two libraries for read DHT11:
DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library
Adafruit Unified Sensor Lib: https://github.com/adafruit/Adafruit_Sensor
Shopping List
Amount | Label | Part Type |
---|---|---|
1 | C1 | 100nF/16V Ceramic Capacitor |
1 | C2 | 470μF/20V Electrolytic Capacitor |
1 | Led1 | Red Led |
1 | Led2 | Yellow Led |
1 | Led3 | Blue Led |
1 | Led4 | Green Led |
1 | Arduino | Arduino |
4 | R1,R2,R3,R4 | 1.5kΩ Resistor |
2 | R5,R6,R7 | 10kΩ Resistor |
1 | D1 | 1N4148 Small signal Diode |
1 | DHT11 | DHT11 Humitidy and Temperature Sensor |
1 | U1 | ESP8266 WiFi Module |
Circuit
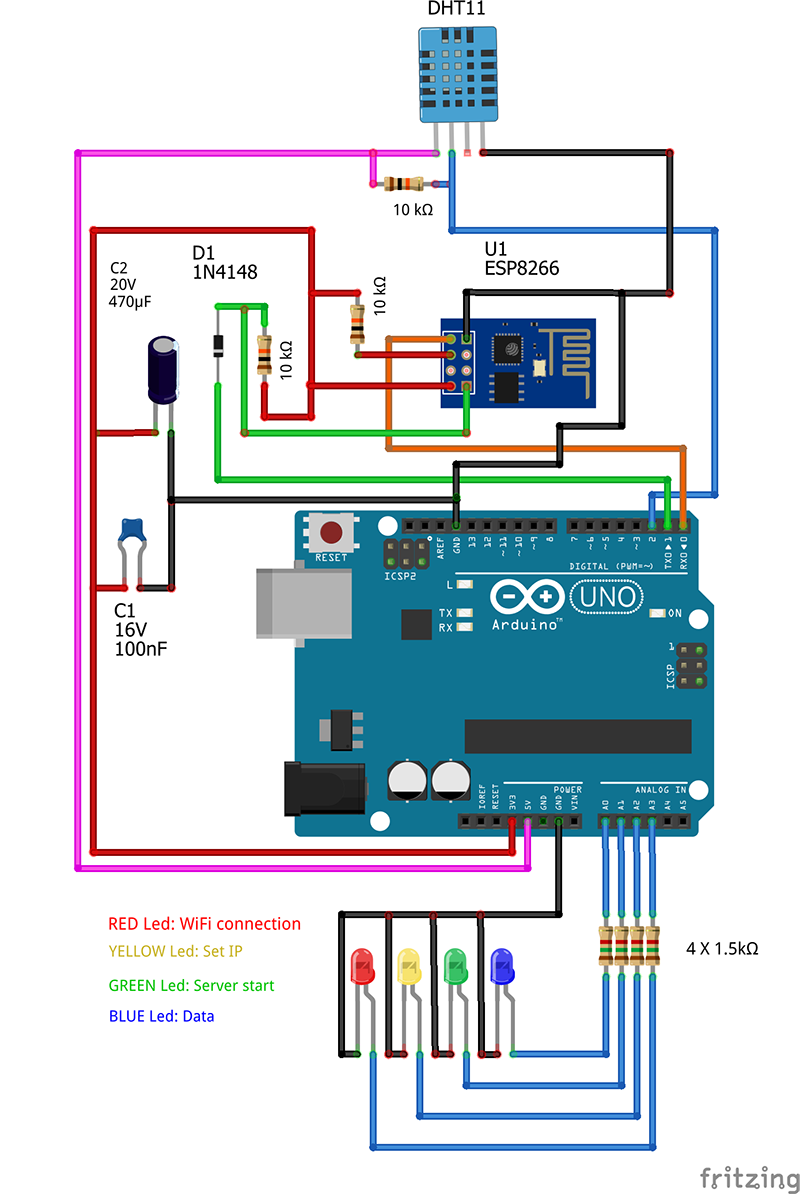
Code
WARNING! Before Upload Sketch to Arduino please remove RX and TX (Pin0 and Pin1) connections from Arduino. After Upload Sketch to Arduino insert this connections again and press Arduino Reset button.
/* Version: 1.3
www.arduinoclub.net/dht11-wifi-humidity-and-temperature.html
REQUIRES the following Arduino libraries:
- DHT Sensor Library: https://github.com/adafruit/DHT-sensor-library
- Adafruit Unified Sensor Lib: https://github.com/adafruit/Adafruit_Sensor
*/
#include <avr/pgmspace.h>
#include "DHT.h"
#define DHTPIN 2 // Digital pin connected to the DHT sensor
#define DHTTYPE DHT11 // DHT 11
#define tx_BufferSize 100
#define tempCharBufferSize 50
char *ssid = "YOUR_SSID"; // SSID
char *ssidPassword = "YOUR_SSID_PASSWORD"; // SSID Password
char *internalIp = "192.168.1.14"; // Internal IP for ESP 8266
char *atCwjab = "AT+CWJAP=\"";
char *atCwjab2 = "\",\"";
char *dq = "\"";
char *atcpsta = "AT+CIPSTA=\"";
char *atcpsnd = "AT+CIPSENDEX=";
char *atcomma = ",";
char tempChar[tempCharBufferSize];
char *splitWithN;
char tx_Buffer[tx_BufferSize];
int count;
char *cmd; // 1=connectToWifi, 2=setCipsta, 3=setCipmux, 4=startCipserver
char delimiterSpace[] = " ";
char delimiterN[] = "\n";
String espResponse;
int str_len;
char requestID[2] = "\0";
char *httpGetData;
const int answerWaitTime = 100;
char humidity[6] = "\0";
char tC[6] = "\0";
char tF[6] = "\0";
// Messages PROGMEM!!!!!
const char messageHttpHeader[] PROGMEM = "HTTP/1.1 200 OK\r\n\r\n";
const char messageOk[] PROGMEM = "OK|";
const char seperator[] PROGMEM = "|";
const char atCipCLose[] PROGMEM = "AT+CIPCLOSE=";
const char atCwMode3[] PROGMEM = "AT+CWMODE=3";
const char atCipMux1[] PROGMEM = "AT+CIPMUX=1";
const char atCipServer1[] PROGMEM = "AT+CIPSERVER=1,80"; // Connection port (Default 80). If change port from here please power of and power on Arduino after upload sketch. need change port number from app settings too
// Initialize DHT sensor.
// Note that older versions of this library took an optional third parameter to
// tweak the timings for faster processors. This parameter is no longer needed
// as the current DHT reading algorithm adjusts itself to work on faster procs.
DHT dht(DHTPIN, DHTTYPE);
void setup() {
pinMode(A3, OUTPUT); // Wifi connection LED
pinMode(A2, OUTPUT); // Set IP LED
pinMode(A1, OUTPUT); // Start server Led
pinMode(A0, OUTPUT); // Data Led
dht.begin();
Serial.begin(115200);
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo only
}
espResponse = sendToWifi("AT", true); // Send AT to Esp
if (find(espResponse, "OK")) { // if get OK
cleanTxBuffer();
strcat_P(tx_Buffer, atCwMode3);
espResponse = sendToWifi(tx_Buffer, true); // Set Esp CWMODE to 3
if (find(espResponse, "OK")) { // if set to CWMODE
cleanTxBuffer();
strcat(tx_Buffer, atCwjab);
strcat(tx_Buffer, ssid);
strcat(tx_Buffer, atCwjab2);
strcat(tx_Buffer, ssidPassword);
strcat(tx_Buffer, dq);
cmd = "1";
espResponse = sendToWifi(tx_Buffer, false); // Try connect to SSID
}
}
}
void loop() {
if ((Serial.available() > 0)) {
digitalWrite(A0, HIGH);
espResponse = "";
espResponse = Serial.readString();
delay(200); // wait for the serial buffer to fill up (read all the serial data)
if (find(espResponse, "+IPD")) { // We have HTTP connection request
str_len = espResponse.length();
char char_array[str_len];
espResponse.toCharArray(char_array, str_len);
espResponse = "";
splitWithN = strtok(char_array, delimiterN);
count = 0;
while (splitWithN != NULL) {
if (count == 0) {
requestID[0] = *(splitWithN);
}
if (count == 2) {
httpGetData = splitWithN;
}
splitWithN = strtok(NULL, delimiterN);
count++;
}
// Read DHT11
// Reading temperature or humidity takes about 250 milliseconds!
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor)
float h = dht.readHumidity();
// Read temperature as Celsius (the default)
float t = dht.readTemperature();
// Read temperature as Fahrenheit (isFahrenheit = true)
float f = dht.readTemperature(true);
// Check if any reads failed and exit early (to try again).
if (isnan(h) || isnan(t) || isnan(f)) {
//Serial.println(F("Failed to read from DHT sensor!"));
return;
}
// Compute heat index in Fahrenheit (the default)
float hif = dht.computeHeatIndex(f, h);
// Compute heat index in Celsius (isFahreheit = false)
float hic = dht.computeHeatIndex(t, h, false);
// /Read DHT11
if (isDigit(requestID[0])) {
cleanTempChar();
strcat_P(tempChar, messageHttpHeader);
strcat_P(tempChar, messageOk);
//dtostrf(floatVar, minStringWidthIncDecimalPoint, numVarsAfterDecimal, charBuf);
dtostrf(h, 4, 2, humidity);
strcat(tempChar, humidity);
strcat_P(tempChar, seperator);
dtostrf(t, 4, 2, tC);
strcat(tempChar, tC);
strcat_P(tempChar, seperator);
dtostrf(f, 4, 2, tF);
strcat(tempChar, tF);
sendData(requestID, tempChar);
}
}
} else {
digitalWrite(A0, LOW);
}
if (cmd != "") {
if (cmd == "1") {
if (find(espResponse, "OK")) {
cmd = "";
digitalWrite(A3, HIGH);
cleanTxBuffer();
strcat(tx_Buffer, atcpsta);
strcat(tx_Buffer, internalIp);
strcat(tx_Buffer, dq);
cmd = "2";
espResponse = sendToWifi(tx_Buffer, false); // Sets the IP Address of the ESP8266 Station
}
}
if (cmd == "2") {
if (find(espResponse, "OK")) {
cmd = "";
digitalWrite(A2, HIGH);
cmd = "3";
cleanTxBuffer();
strcat_P(tx_Buffer, atCipMux1);
espResponse = sendToWifi(tx_Buffer, false); // Configure ESP for multiple connections
}
}
if (cmd == "3") {
if (find(espResponse, "OK")) {
cmd = "";
cmd = "4";
cleanTxBuffer();
strcat_P(tx_Buffer, atCipServer1);
espResponse = sendToWifi(tx_Buffer, false); // Turn on server on port 80
}
}
if (cmd == "4") {
if (find(espResponse, "OK")) {
cmd = "";
digitalWrite(A1, HIGH);
}
}
}
}
/*
Name: sendToWifi
Description: Function used to send data to ESP8266.
Params: atCommand - the data/command to send; getAnswer - get return data from ESP8266 (true = yes, false = no)
Returns: The response from the esp8266 (if there is a reponse and if getAnswer = true)
*/
String sendToWifi(char* atCommand, boolean getAnswer) {
espResponse = "";
Serial.println(atCommand);
if (getAnswer == true) {
delay(answerWaitTime);
if (Serial.available() > 0) {
while (Serial.available()) {
espResponse = Serial.readString();
}
}
}
return espResponse;
}
/*
Name: find
Description: Function used to match two string
Params: s - search in value
Returns: true if match else false
*/
boolean find(String s, String value) {
if (s.indexOf(value) >= 0) {
return true;
} else {
return false;
}
}
void sendData(char* reqID, char* dataStr) {
char len[3] = "\0";
char len_Value = strlen(dataStr);
if (len_Value < 10 ) {
len[0] = (len_Value % 10) + 48;
}
if (len_Value >= 10 ) {
len[0] = ((len_Value / 10) % 10) + 48;
len[1] = (len_Value % 10) + 48;
}
cleanTxBuffer();
strcat(tx_Buffer, atcpsnd);
strcat(tx_Buffer, reqID);
strcat(tx_Buffer, atcomma);
strcat(tx_Buffer, len);
sendToWifi(tx_Buffer, true);
delay(100); // If you live answer problems increase this value
if (find(espResponse, ">")) {
sendToWifi(dataStr, false);
delay(250);
cleanTxBuffer();
strcat_P(tx_Buffer, atCipCLose);
strcat(tx_Buffer, reqID);
sendToWifi(tx_Buffer, false);
} else {
cleanTxBuffer();
strcat_P(tx_Buffer, atCipCLose);
strcat(tx_Buffer, 5);
sendToWifi(tx_Buffer, false);
}
}
void cleanTxBuffer() {
for (count = 0; count < tx_BufferSize; count ++) {
tx_Buffer[count] = '\0';
}
}
void cleanTempChar() {
for (count = 0; count < tempCharBufferSize; count ++) {
tempChar[count] = '\0';
}
}